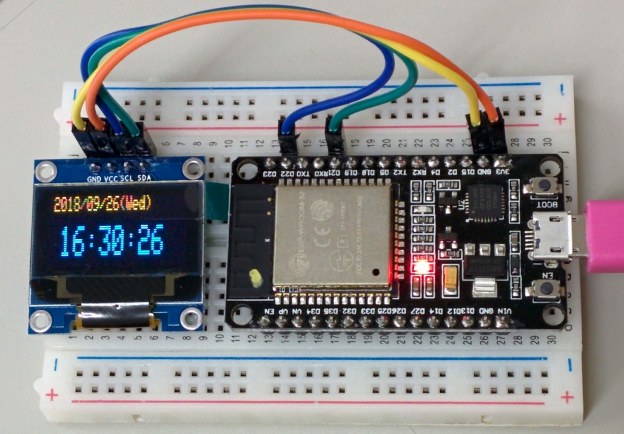
目的
SSD1306に時刻を表示するプログラム。
ESP8266と違って、SCL,SDAを探すも一苦労。
- I2C0 – SDA,SCL = 21,22
**2019/9/2 注意:Adafruit_SSD1306関数の引数順番変更により、関連プログラムが影響する。
もともと次のような1行ものが、
// Adafruit_SSD1306 display(OLED_RESET);
次の数行に変わる。
// OLED Setting
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
// Declaration for an SSD1306 display connected to I2C (SDA, SCL pins)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
なぜ互換性ないの引数順にするね?!どうしてもしたいならば、コンパイルエラーを出す方法で、修正を促す。
以前のプログラムが再検証するところ、エラーがなし、書き込みも成功、しかしOLEDが表示ない!困った。
ハードウェア故障?ソフトウェアバージョン問題?色々と検討して、数日無駄の末、やっと引数順変わったとわかった。Adafruitひどい!
ハードウェア
「DOIT ESP32 DEVKIT V1」が幅が広いから、ブレッドボードは片側しか空いてない。結線は、片側で間にってよかった。
「ESPDUINO-32」の場合も動作する
ソフトウェア
参考1のそのまま
#include <WiFi.h> #include <time.h> #include <SPI.h> #include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> // OLED Setting #define OLED_RESET 4 Adafruit_SSD1306 display(OLED_RESET); #if (SSD1306_LCDHEIGHT != 32) #error("Height incorrect, please fix Adafruit_SSD1306.h!"); #endif // WiFi Setting #define WIFI_SSID "uislab003" #define WIFI_PASSWORD "nihao12345" #define JST 3600*9 void setup() { Serial.begin(115200); delay(100); Serial.print("\n\nReset:\n"); display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // initialize with the I2C addr 0x3C (for the 128x32) // Clear the buffer. display.clearDisplay(); display.setTextColor(WHITE); // WiFi starting drawLog("WiFi connecting..."); WiFi.begin(WIFI_SSID, WIFI_PASSWORD); while(WiFi.status() != WL_CONNECTED) { Serial.print('.'); delay(500); } Serial.println(); Serial.printf("Connected, IP address: "); Serial.println(WiFi.localIP()); drawLog("WiFi connected!"); // NTP start configTime( JST, 0, "ntp.nict.jp", "ntp.jst.mfeed.ad.jp"); delay(1000); } void loop() { time_t t; struct tm *tm; static const char *wd[7] = {"Sun","Mon","Tue","Wed","Thr","Fri","Sat"}; char rdate[30], rtime[30]; t = time(NULL); tm = localtime(&t); Serial.printf(" %04d/%02d/%02d(%s) %02d:%02d:%02d\n", tm->tm_year+1900, tm->tm_mon+1, tm->tm_mday, wd[tm->tm_wday], tm->tm_hour, tm->tm_min, tm->tm_sec); sprintf(rdate, " %04d/%02d/%02d(%s)", tm->tm_year+1900, tm->tm_mon+1, tm->tm_mday, wd[tm->tm_wday]); sprintf(rtime, " %02d:%02d:%02d", tm->tm_hour, tm->tm_min, tm->tm_sec); drawClock(rdate, rtime); delay(1000 - millis()%1000); } void drawClock(const char* rdate, const char* rtime) { display.clearDisplay(); display.setCursor(0,0); display.setTextSize(1); drawText(rdate); display.setCursor(0,13); display.setTextSize(2); drawText(rtime); display.display(); delay(1); } void drawText(const char* text) { for (uint8_t i=0; i < strlen(text); i++) { display.write(text[i]); } } void drawLog(const char* msg) { display.clearDisplay(); display.setCursor(0,0); display.setTextSize(1); drawText(msg); display.display(); delay(1); }
参考
- https://qiita.com/nori-dev-akg/items/bbe269d1d1bf1826532a