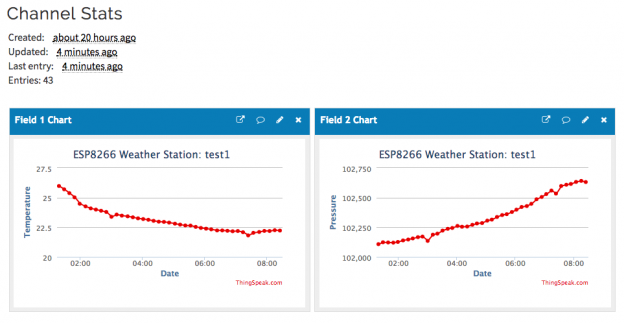
数回JSON関連の実験をしたが、いざTinyWebDBのAPIの実験を始まると、また引っかかるところが多い。
色々と検索してところ、ThingspeakのAPIサンプルが見つかったので、ちょっと曲がり道して試すことに。
センサーの温度と気圧をThingspeakにアップして、動きを見て見る。
まずThingspeakのアカウントを申請して、THINGSPEAK_API_KEYを取得する。
https://thingspeak.com/users/sign_up
次はサンプルを見ながら、プログラミング。
#include <Adafruit_BMP280.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #define OLED_RESET 0 // GPIO0 Adafruit_SSD1306 OLED(OLED_RESET); #define BMP_SCK 13 #define BMP_MISO 12 #define BMP_MOSI 11 #define BMP_CS 10 Adafruit_BMP280 bmp; // I2C //Adafruit_BMP280 bmp(BMP_CS); // hardware SPI //Adafruit_BMP280 bmp(BMP_CS, BMP_MOSI, BMP_MISO, BMP_SCK); #include <ESP8266WiFi.h> #include <ESP8266HTTPClient.h> /*************************** * Begin Settings **************************/ const char* host = "http://api.thingspeak.com"; const char* THINGSPEAK_API_KEY = "***********"; // Update every 600 seconds = 10 minutes. Min with Thingspeak is ~20 seconds const int UPDATE_INTERVAL_SECONDS = 600; //needed for library #include <DNSServer.h> #include "WiFiManager.h" //https://github.com/tzapu/WiFiManager void configModeCallback (WiFiManager *myWiFiManager) { Serial.println("Entered config mode"); Serial.println(WiFi.softAPIP()); //if you used auto generated SSID, print it Serial.println(myWiFiManager->getConfigPortalSSID()); } /*************************** * End Settings **************************/ void setup() { OLED.begin(); OLED.clearDisplay(); //Add stuff into the 'display buffer' OLED.setTextWrap(false); OLED.setTextSize(1); OLED.setTextColor(WHITE); OLED.setCursor(0,0); delay(10); Serial.begin(115200); delay(10); // We start by connecting to a WiFi network // Connect to WiFi network OLED.println("wifiManager autoConnect..."); OLED.display(); //output 'display buffer' to screen //WiFiManager //Local intialization. Once its business is done, there is no need to keep it around WiFiManager wifiManager; //reset settings - for testing //wifiManager.resetSettings(); //set callback that gets called when connecting to previous WiFi fails, and enters Access Point mode wifiManager.setAPCallback(configModeCallback); //fetches ssid and pass and tries to connect //if it does not connect it starts an access point with the specified name //here "AutoConnectAP" //and goes into a blocking loop awaiting configuration if(!wifiManager.autoConnect()) { Serial.println("failed to connect and hit timeout"); //reset and try again, or maybe put it to deep sleep ESP.reset(); delay(1000); } // Print the IP address OLED.print("http://"); OLED.print(WiFi.localIP()); OLED.println("/"); OLED.println("WiFi connected"); OLED.display(); //output 'display buffer' to screen //if you get here you have connected to the WiFi Serial.println("connected...yeey :)"); if (!bmp.begin(0x76)) { OLED.println("Could not find BMP180 or BMP085 sensor at 0x77"); OLED.display(); //output 'display buffer' to screen while (1) {} } } void loop() { // read values from the sensor float pressure = bmp.readPressure(); float temperature = bmp.readTemperature(); // We now create a URI for the request String url = host; url += "/update?api_key="; url += THINGSPEAK_API_KEY; url += "&field1="; url += String(temperature); url += "&field2="; url += String(pressure); Serial.print("Requesting URL: "); Serial.println(url); HTTPClient http; Serial.print("[HTTP] begin...\n"); // configure targed server and url http.begin(url); Serial.print("[HTTP] GET...\n"); // start connection and send HTTP header int httpCode = http.GET(); if(httpCode == HTTP_CODE_OK) { String buffer = http.getString(); Serial.println(buffer); } Serial.println("closing connection"); // Go back to sleep. If your sensor is battery powered you might // want to use deep sleep here delay(1000 * UPDATE_INTERVAL_SECONDS); }
こちら問題なくデータの蓄積ができた。